You can download YouTube videos with the NetVideoHunter Firefox add-on. After installing the add-on, you can download a YouTube video by clicking the icon.
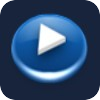
By default the add-on downloads the best available quality from YouTube, that is very convenient.
The downloaded file has an mp4 extension. Using the libav command line tool avconv, you can extract the audio without transcoding. This way the process is very fast and the audio quality remains the same:
avconv -i testvideo.mp4 -codec copy -vn testaudio.m4a
The -codec copy option makes sure the audio is extracted without conversion an the -vn option excludes the video being written to the output file.
To install avconv on Debian:
sudo apt-get install libav-tools